
Angular Unit Testing
Umar Zai
Deep Dive into Angular Unit Testing: Tools and Techniques
Introduction to Angular Unit Testing
When we talk about software development, ensuring the quality and reliability of code is paramount. This is where unit testing, especially Angular Unit Testing, comes into play. Unit tests are designed to independently validate each part of the software by isolating it from its external dependencies.
In the realm of Angular, unit testing focuses primarily on checking individual functions, components, services, and other building blocks of the application. These tests ensure that, under given inputs, your code produces the expected output. A successful unit test indicates that the smaller, isolated parts of your codebase function as expected, which is essential for the overall health and functionality of larger applications.
Angular Unit Testing is enhanced by frameworks and tools designed specifically for the job. Among the most notable are:
- Jasmine: At the heart of Angular Unit Testing is Jasmine, a behavior-driven development framework for testing JavaScript code. It provides developers with a clean and readable syntax to define tests, often making use of describe and it blocks to structure and label these tests. With Jasmine, Angular Unit Testing becomes more intuitive, allowing developers to easily specify conditions and expected results.
- Karma: Complementing Jasmine in the Angular Unit Testing ecosystem is Karma. It’s not just any test runner. Karma offers the capability to launch actual browsers, be it Chrome, Firefox, or others. This ensures that Angular applications are tested in real-world conditions. After running the tests, Karma diligently reports the results, allowing developers to quickly spot and rectify any issues. When integrated properly, Karma makes Angular Unit Testing a smooth and streamlined process.
Setting Up Jasmine and Karma
Angular Unit Testing is undeniably crucial in the modern development landscape. While the importance of unit tests can’t be overstated, the tools that facilitate these tests are equally vital. Jasmine, as a behavior-driven development framework, and Karma, a test runner, are two such pivotal tools. Thankfully, for developers working on Angular projects, setting up these tools is a breeze, thanks to the Angular CLI.
The Angular CLI Advantage
One of the most remarkable features of Angular’s ecosystem is the Angular CLI (Command-Line Interface). The CLI is not just a tool; it’s a developer’s best friend, offering a plethora of commands to scaffold, build, test, and deploy Angular applications. When you kickstart an Angular project using the CLI, it is pre-configured for unit testing, embedding both Jasmine and Karma seamlessly. This decision by the Angular team is a testament to the emphasis they place on testing, and it significantly reduces the friction in starting with Angular Unit Testing.
Essential Files for Testing
Within an Angular project initiated by the CLI, there are certain key files and conventions that pertain directly to testing:
- karma.conf.js: This is the central configuration file for Karma. It dictates how Karma behaves and runs the tests. Developers can specify browsers, reporters, and other settings here. For example, if you prefer running tests on Chrome or want to integrate continuous integration processes, this file is where such configurations reside. While the default settings cater to most needs, the flexibility offered ensures that Angular Unit Testing adapts to a variety of project requirements.
- test.ts: Often termed as the “entry point” for Angular Unit Testing, test.ts is crucial. It’s responsible for initializing the Angular testing environment and bootstrapping the tests. Before any test runs, test.ts ensures that the necessary modules, such as the Angular-specific testing modules, are in place. This systematic initialization guarantees that every test operates within a consistent environment.
- *.spec.ts files: Following a widely-adopted naming convention, .spec.ts files are the heartbeats of Angular Unit Testing. Each spec file corresponds to a particular unit that’s being tested—be it a component, service, directive, or pipe. For instance, if you have a component named user.component.ts, its corresponding test file would ideally be user.component.spec.ts. This convention makes it easier to locate tests and understand the codebase’s testing coverage. Every .spec.ts file typically contains a series of test cases, written using Jasmine’s syntax, that validate the functionality of its corresponding unit.
Expanding on Angular Unit Testing
While the foundation is set up by default, the real challenge and artistry lie in crafting meaningful tests. Angular Unit Testing requires developers to think from a user’s perspective, anticipate edge cases, and validate both the expected and unexpected. Jasmine and Karma, in this endeavor, act as the trusted allies. Jasmine provides the syntax, constructs, and assertion mechanisms, while Karma ensures that these tests run consistently across different environments.
As you delve deeper into Angular development, you’ll invariably find scenarios that need advanced configurations or integrations, be it incorporating third-party libraries or tweaking settings for performance optimizations. It’s comforting to know that Jasmine and Karma, while being easy for beginners, offer the depth and flexibility that seasoned developers appreciate.
Writing a Basic Test
Delving into the world of Angular Unit Testing reveals a fascinating landscape where developers can validate their code, ensuring that each individual part of their application functions precisely as intended. For those new to the realm of testing, or specifically to Angular Unit Testing, understanding the foundational principles and structures is pivotal. Among these foundational elements are the describe and it blocks, which are integral to structuring and defining tests.
The Essence of Jasmine’s Syntax
Before we delve deep into the constructs, it’s essential to realize that the syntax we employ in Angular Unit Testing, especially the describe and it blocks, stems from Jasmine. Jasmine, being a behavior-driven development framework, is crafted to make tests readable and representative of the intended behavior. The goal is to make tests almost like readable sentences that describe the expected functionality of a unit.
describe Block: Setting the Stage
Think of the describe block as the title or heading of a chapter in a book. It gives context, setting the stage for what’s to come. In the domain of Angular Unit Testing, the describe block encapsulates a set of tests pertaining to a specific functionality or unit, be it a component, service, or any other element.
it Block: The Individual Test Cases
Within the overarching context set by describe, the individual test cases are defined using the it blocks. If describe is the chapter heading, then it blocks are the various sections or subsections within that chapter.
Bringing It All Together in Angular Unit Testing
When you merge the describe and it blocks, you lay down a structured, readable, and comprehensive testing suite. As you craft more tests and your application grows, these blocks become the bedrock of your Angular Unit Testing strategy. They help in organizing tests, ensuring that each function, component, or service is validated against its intended behavior.
But writing a basic test is merely the tip of the iceberg. As developers dive deeper into Angular Unit Testing, they encounter scenarios that require mocking dependencies, handling asynchronous operations, or testing user interactions. Yet, the foundational understanding of the describe and it blocks remains crucial.
Test Components, Services, and Directives
Testing in Angular is not a one-size-fits-all process; it’s a multi-faceted endeavor that extends to various aspects of the application. The breadth and depth of Angular Unit Testing are evident when you consider that not just services, but also components and directives, need to be tested. Each of these units has a unique role within an Angular application, and therefore, each requires specialized approaches to testing.
Testing Components: The Building Blocks
Components are the primary building blocks of Angular applications. They control views (HTML templates) and handle data. Angular Unit Testing for components often involves checking if the component is created, if it renders the correct data, or if it responds correctly to user interactions.
Angular offers a specialized testing module, TestBed, to create a dynamic Angular testing module where you can declare your components and simulate the environment they run in.
Testing Services: The Logic Centers
Services in Angular serve as a reusable logic center. Angular Unit Testing for services often includes testing HTTP requests, method return types, or observable streams.
Services can usually be instantiated directly, but you can also use Angular’s TestBed for more complex scenarios.
Testing Directives: The Behavior Modifiers
Directives modify the behavior of DOM elements. Testing directives often involves checking if the DOM changes as expected when the directive is applied. Like components, directives are also typically tested using TestBed.
The Unified Strategy in Angular Unit Testing
Despite the differences between components, services, and directives, the underlying strategy of Angular Unit Testing remains the same: isolate the unit of work, simulate its behavior, and assert that it functions as expected.
Asynchronous Testing
Asynchronous operations are inherent in modern web applications, especially in scenarios like HTTP requests, timers, and various other background tasks. Angular, being a framework tailored for building sophisticated applications, naturally deals with a lot of asynchronous code. However, asynchronous code can be tricky to test because of its non-linear execution. To address this challenge, Angular Unit Testing offers a suite of utilities, like fakeAsync, tick, and async, designed to streamline and simplify the testing of asynchronous operations.
fakeAsync and tick: Controlling Time
The fakeAsync function is used to write tests that look and feel synchronous but test asynchronous code. Within the fakeAsync block, the tick function is used to simulate the passage of time, essentially allowing you to fast-forward or control time.
async: Real Asynchronous Testing
While fakeAsync and tick give you a pseudo-synchronous way to test asynchronous operations, sometimes you want the test to run in a real asynchronous fashion. This is where the async utility comes into play.
The async function is used to wrap any test that has asynchronous operations and makes sure the test environment waits for all asynchronous tasks to complete before ending the test.
Mocking Dependencies
Testing in Angular often requires us to simulate real-world scenarios without causing side effects or relying on external factors. For instance, when unit testing a component or service, you might not want to make actual HTTP requests or access real services. This is where mocking comes into play. In the context of Angular Unit Testing, mocking refers to substituting real implementations of dependencies with controlled, simulated versions. This ensures tests are isolated, faster, and more reliable. Angular’s TestBed plays a pivotal role in facilitating this process.
Why Mock?
- Isolation: Unit tests should focus on testing a ‘unit’ in isolation. If your component or service interacts with another service, you want to ensure you’re only testing the behavior of the unit in question, not the service it interacts with.
- Control: Mocks provide controlled responses to function calls, allowing you to simulate various scenarios.
- Speed: Real dependencies, like making HTTP requests, can slow down tests. Mocks are instantaneous, making your tests faster.
- Safety: Avoid side effects. For instance, you wouldn’t want your tests to make actual HTTP POST requests and create data on a server.
Using TestBed to Mock Services
One of the most common use-cases for mocking in Angular Unit Testing is when a component relies on a service. Rather than use the actual service, you can provide a mock version of it.
Mocking HTTP Requests
Angular’s HttpClientTestingModule and HttpTestingController allow you to mock HTTP requests. This ensures your tests don’t rely on actual API endpoints and can simulate different responses.
Running and Reporting Tests
Once you’ve written your unit tests, the next critical steps in the Angular Unit Testing lifecycle are to execute these tests and analyze the outcomes. Running tests helps ensure your application’s functionality remains intact as you add new features or make changes. Reporting, on the other hand, provides insights into test results, helping teams pinpoint issues, enhance code quality, and maintain documentation of application health over time.
Best Practices
Ensuring the reliability and maintainability of tests is vital for the sustainability of large-scale applications. Over time, as applications grow and evolve, the importance of adhering to testing best practices becomes more pronounced. Let’s dive deeper into some of the best practices for Angular Unit Testing, understanding the rationale behind each.
- Write Small and Focused Tests
Rationale:
- Clarity: Smaller tests with a specific focus are easier to understand. When someone looks at a test, they should immediately grasp what’s being tested and what the expected behavior is.
- Troubleshooting: When a test fails, it should quickly point out what part of the codebase has an issue. Broad tests can make it challenging to identify the exact problem.
Implementation:
- Each test should validate only one specific behavior or function.
- Use descriptive test names that convey the purpose of the test.
- Mock External Dependencies
Rationale:
- Isolation: Unit tests should test a ‘unit’ in isolation, ensuring the unit’s behavior is correct regardless of external factors.
- Performance: Mocks are faster. They eliminate the overhead of initializing real services or making actual HTTP requests.
Implementation:
- Use tools like TestBed to provide mock versions of services or components.
- Utilize Angular’s HttpClientTestingModule to mock HTTP requests.
- Test Public Interfaces, Not Internal Details
Rationale:
- Maintainability: Internal implementation can change over time, but public interfaces usually remain stable. Tests should not break just because the internal logic is refactored.
- Relevance: End-users interact with public interfaces, not the nitty-gritty details. Thus, ensuring these interfaces work correctly is of utmost importance.
Implementation:
- Avoid testing private methods directly. Instead, test the public methods that call them.
- Don’t rely on the internal state of a component or service. Test its output or its public methods.
- Keep Tests Independent from Each Other
Rationale:
- Reliability: If one test fails, it shouldn’t cause a cascade of failures in unrelated tests.
- Predictability: Tests should produce the same results regardless of the order they’re run in.
Implementation:
- Ensure each test sets up its own environment.
- Avoid sharing state between tests. Reinitialize any shared resources in a beforeEach block.
- Maintain a Good Ratio of Unit Tests to Integration Tests
Rationale:
- Coverage vs. Complexity: While unit tests ensure individual components function correctly in isolation, integration tests validate the interaction between these components. Both are crucial, but unit tests are generally quicker and easier to write and maintain.
- Feedback Loop: Unit tests provide rapid feedback during development, while integration tests ensure the entire system works in harmony.
Implementation:
- For core functionalities and complex logic, ensure thorough unit test coverage.
- Identify critical interaction points in your application and write integration tests for those.
- Ensure integration tests cover scenarios where multiple units interact, especially if those interactions involve third-party libraries or external systems.
Conclusion
In the realm of Angular Unit Testing, adhering to best practices is paramount for achieving test reliability. Moreover, these practices also lend greater maintainability and clarity to your test suite. As Angular Unit Testing serves as the bedrock for safeguarding the persistent health and quality of your application, dedicating time to craft well-structured tests and steadfastly sticking to these practices is a wise and invaluable investment. Through the disciplined approach of Angular Unit Testing, developers can ensure the longevity and robustness of their applications.
About Remote IT Professionals
Remote IT Professionals is devoted to helping remote IT professionals improve their working conditions and career prospects.
We are a virtual company that specializes in remote IT solutions. Our clients are small businesses, mid-sized businesses, and large organizations. We have the resources to help you succeed. Contact us for your IT needs. We are at your service 24/7.
Posted on: August 24, 2023 at 6:01 am
Best Website Design Companies Houston, Texas
Umar Zai  November 22, 2023
Profiles and Demonstrated Record: Best Website Design Companies in Houston, Texas Houston, Texas, stands as a burgeoning hub for innovation…
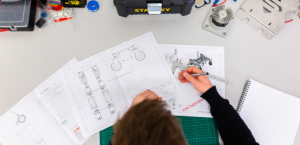
Best Web Design Companies in El Paso
Umar Zai  
Leading in the List: Best Web Design Companies in El Paso, Texas. El Paso is a vibrant city known for…
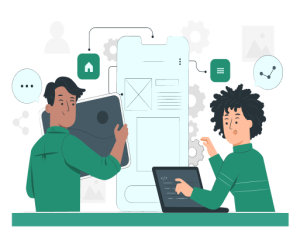
Website Designers San Antonio
Umar Zai  
Ultimate Selection: Best Website Designers in San Antonio, Texas The best website designers in San Antonio, Texas, are highly esteemed…
Cloud Computing Startup Companies
Umar Zai  November 13, 2023
Exploring the Landscape of Popular Cloud Computing Startup Companies Cloud computing has revolutionised the way businesses operate, providing scalable and…
WordPress Blog PlugIns
Umar Zai  
Exploring the best WordPress blog plugins for maximum impact In the dynamic world of blogging, the choice of the best…
AI Language Models
Umar Zai  
Exploring Progress and Obstacles: Delving into the Influence of AI Language Models on Society In the ever-evolving landscape of artificial…
Latest Tweet
No tweets found.