Reactive Programming
Umar Zai
Implementing Reactive Programming with Java 8 Streams
Reactive Programming is a programming paradigm that allows for a more responsive and efficient way of processing data streams. With its ability to handle real-time events and data streams, Reactive Programming has gained a lot of popularity in recent years, especially in the field of web development. Java 8, on the other hand, introduced a new and powerful Stream API that allows for functional programming and stream processing. By combining the principles of Reactive Programming with the capabilities of Java 8 Streams, developers can build reactive applications that are scalable, resilient, and efficient.
In this article, we will explore the world of Reactive Programming with Java 8 Streams. We will start by understanding the principles and concepts of Reactive coding and how they apply to Java 8 Streams. We will then dive into the benefits of Reactive coding with Java 8 Streams and how to set up a Reactive Programming environment. We will cover various topics such as applying Reactive coding to data streams, parallel execution, testing, and best practices. Finally, we will explore the challenges and future of Reactive Programming with Java 8 Streams and demonstrate how to implement Reactive coding in real-world Java applications. So, let’s get started and see how to leverage the power of Reactive coding with Java 8 Streams!
Understanding Reactive Programming Principles and Concepts
Reactive Programming is a programming paradigm that is focused on processing data streams in a responsive and efficient manner. It allows developers to handle real-time events and data streams with ease. In Reactive Programming, data flows through a pipeline of functions that can be composed and transformed. This allows for a more modular and reusable codebase. Java 8 Streams, with their functional programming capabilities, are an excellent fit for Reactive coding. The key principles of Reactive Programming include the ability to handle asynchronous data streams, a focus on event-driven programming, and the ability to react to changes in real-time. Reactive coding allows for efficient use of resources by only processing data as needed, rather than continuously polling or processing all data at once.
In Java 8 Streams, Reactive Programming can be achieved by using functional programming techniques such as mapping, filtering, and reducing. The use of Lambdas and method references enables concise and expressive code that is easy to read and maintain.
The Benefits of Reactive Programming with Java 8 Streams
Reactive Programming with Java 8 Streams offers several benefits to developers. One of the key benefits is the ability to handle asynchronous data streams efficiently. Reactive Programming allows developers to process data as it becomes available, rather than processing all data at once, which can be resource-intensive. Another benefit of Reactive Programming is the ability to handle real-time events and data streams with ease. This is particularly useful in applications such as financial trading systems, real-time analytics, and IoT applications, where data is constantly changing. Java 8 Streams, with their functional programming capabilities, allow for a more modular and reusable codebase. This makes it easier to build and maintain complex applications.
Reactive Programming also promotes a more event-driven programming style, which can help to improve the scalability and responsiveness of applications. By reacting to events as they happen, rather than polling for changes, applications can be more efficient and responsive. Finally, Reactive Programming can help to improve the overall user experience of applications by providing a more responsive and seamless experience.
Setting up a Reactive Programming Environment with Java 8 Streams
Setting up a Reactive coding environment with Java 8 Streams is a straightforward process. To get started, developers need to ensure that they have a suitable IDE and the latest version of Java 8 installed on their system. The next step is to include the necessary libraries and dependencies for Reactive coding. This includes libraries such as RxJava, Reactor, and Akka Streams. These libraries provide additional tools and features for Reactive Programming, such as back-pressure handling and reactive streams.
Developers can then begin building reactive applications by creating and manipulating streams of data using Java 8’s Stream API. They can use functional programming techniques such as mapping, filtering, and reducing to transform data streams and apply operations to them. In addition to the standard Java 8 libraries, developers can also use third-party libraries and frameworks such as Spring Boot and Vert.x to build reactive applications. These frameworks provide additional features such as dependency injection, REST APIs, and web-sockets.
Understanding Stream API in Java 8 and its Reactive Capabilities
Java 8 introduced the Stream API, which is a powerful tool for processing collections of data. Streams allow developers to perform functional-style operations on data, such as filtering, mapping, and reducing. These operations can be performed in a single pass over the data, making them more efficient and expressive. The Stream API in Java 8 also has reactive capabilities, making it well-suited for Reactive Programming. Streams can be processed asynchronously, allowing for efficient handling of data streams that arrive at irregular intervals. Streams also support back-pressure handling, which allows for efficient management of data streams in real-time applications.
The functional programming capabilities of the Stream API allow developers to easily compose and transform data streams, making it a powerful tool for Reactive Programming. By using Lambdas and method references, developers can write concise and expressive code that is easy to read and maintain.
Applying Reactive Programming to Data Streams with Java 8
Reactive Programming can be applied to data streams with Java 8 using the Stream API. The Stream API provides a powerful set of operations that can be used to manipulate and process data streams in a reactive and efficient manner. Developers can use functional programming techniques such as mapping, filtering, and reducing to transform data streams and apply operations to them. These operations can be performed in a single pass over the data, making them more efficient and expressive.
Additionally, developers can use third-party libraries such as RxJava and Reactor to build reactive applications. These libraries provide additional tools and features for Reactive Programming, such as backpressure handling and reactive streams. By leveraging the capabilities of Java 8 Streams and third-party libraries, developers can build powerful and reactive applications that can handle real-time and streaming data with ease. Reactive Programming with Java 8 Streams provides a powerful and efficient way to handle data streams and build responsive and scalable applications.
Building Reactive Applications with Java 8 Streams and Lambdas
Building Reactive Applications with Java 8 Streams and Lambdas is a powerful and efficient way to handle data streams and build responsive and scalable applications. With the functional programming capabilities of Java 8 Streams and Lambdas, developers can easily compose and transform data streams. Reactive coding with Java 8 Streams and Lambdas involves processing streams of data asynchronously and reacting to events as they occur. By using functional programming techniques such as mapping, filtering, and reducing, developers can transform data streams and apply operations to them in a reactive and efficient manner.
Furthermore, third-party libraries such as RxJava and Reactor can be used to build reactive applications. These libraries provide additional tools and features for Reactive Programming, such as back-pressure handling and reactive streams.
Reactive Programming Best Practices for Java 8 Streams
Reactive Programming with Java 8 Streams requires adherence to best practices to ensure that applications are efficient, scalable, and maintainable. One best practice is to use non-blocking I/O and asynchronous processing whenever possible. This enables applications to handle large volumes of data without blocking threads and causing performance issues. Another best practice is to use back-pressure techniques to handle situations where the producer is generating data faster than the consumer can process it. Back-pressure techniques, such as buffering or throttling, enable applications to manage data streams and prevent data loss or resource exhaustion.
Additionally, developers should ensure that they handle errors and exceptions effectively in reactive applications. This includes using techniques such as circuit breakers, timeouts, and retries to handle failures gracefully and prevent cascading failures. Lastly, developers should use reactive libraries and frameworks such as Reactor or RxJava, which provide a set of tools and utilities for building reactive applications.
Testing and Debugging Reactive Programs with Java 8 Streams
Testing and Debugging Reactive Programs with Java 8 Streams can be challenging due to the asynchronous and reactive nature of the programming model. However, there are tools and techniques that can be used to effectively test and debug reactive programs. One technique is to use reactive testing frameworks such as RxJavaTest or ReactorTest, which provide a set of testing utilities for reactive coding. These frameworks enable developers to test reactive streams and verify that the streams are processing data correctly.
Additionally, developers can use debugging tools such as the Java debugger and logging frameworks to help diagnose issues in reactive programs. By using these tools, developers can step through the code and identify issues in the reactive stream.
Common Challenges and Pitfalls of Reactive Programming with Java 8 Streams
Reactive Programming with Java 8 Streams can present challenges and pitfalls that developers should be aware of. One common challenge is dealing with backpressure, which occurs when the producer is generating data faster than the consumer can process it. To handle backpressure, developers can use techniques such as throttling or buffering. Another challenge is ensuring thread safety in a concurrent environment. Reactive coding with Java 8 Streams involves asynchronous and concurrent processing, which can lead to thread safety issues if not handled properly. To ensure thread safety, developers can use techniques such as synchronization, locking, and atomic variables.
Additionally, developers may encounter issues related to debugging and testing reactive programs, as mentioned in the previous section. Effective testing and debugging of reactive programs require specialized tools and techniques.
Combining Reactive Programming with Other Java Technologies
Reactive Programming with Java 8 Streams can be combined with other Java technologies to build powerful and efficient applications. One technology that can be combined with Reactive Programming is Spring Framework. Spring provides a comprehensive set of tools and features for building reactive applications, such as Spring WebFlux and Spring Data R2DBC. Another technology that can be combined with Reactive Programming is Apache Kafka. Kafka is a distributed streaming platform that enables developers to build real-time streaming applications. By using Reactive Programming with Kafka, developers can process Kafka data streams asynchronously and react to events in real-time.
Additionally, developers can use Reactive coding with Java 8 Streams to build microservices with technologies such as Kubernetes and Docker. By leveraging the reactive capabilities of Java 8 Streams, developers can build responsive and scalable microservices that can handle large volumes of data.
Future of Reactive Programming in Java and Java 8 Streams
The future of Reactive coding in Java and Java 8 Streams looks promising. The popularity of Reactive Programming is increasing rapidly due to its ability to handle real-time and streaming data in an efficient and scalable manner. Many developers are already using Java 8 Streams and libraries like RxJava and Reactor to build reactive applications. With the release of Java 9 and newer versions, Java has continued to add features that enhance its capabilities for Reactive Programming. For example, the Flow API introduced in Java 9 provides a standardized way to implement reactive streams and backpressure handling.
Furthermore, many popular frameworks and libraries for building reactive applications, such as Spring Boot and Akka Streams, continue to evolve and integrate with Java 8 Streams. As the demand for real-time and streaming data applications continues to grow, Reactive Programming and Java 8 Streams are likely to remain a popular choice for developers. By leveraging the power of functional programming and reactive streams, Java 8 Streams provide a powerful and efficient way to handle data streams and build responsive and scalable applications.
About Remote IT Professionals
Remote IT Professionals is devoted to helping remote IT professionals improve their working conditions and career prospects.
We are a virtual company that specializes in remote IT solutions. Our clients are small businesses, mid-sized businesses, and large organizations. We have the resources to help you succeed. Contact us for your IT needs. We are at your service 24/7.
Posted on: May 7, 2023 at 8:08 am
Best Website Design Companies Houston, Texas
Umar Zai  November 22, 2023
Profiles and Demonstrated Record: Best Website Design Companies in Houston, Texas Houston, Texas, stands as a burgeoning hub for innovation…
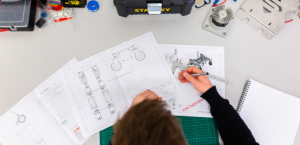
Best Web Design Companies in El Paso
Umar Zai  
Leading in the List: Best Web Design Companies in El Paso, Texas. El Paso is a vibrant city known for…
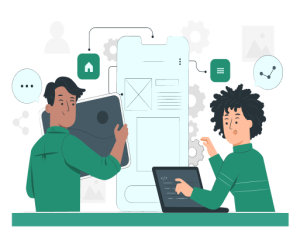
Website Designers San Antonio
Umar Zai  
Ultimate Selection: Best Website Designers in San Antonio, Texas The best website designers in San Antonio, Texas, are highly esteemed…
Cloud Computing Startup Companies
Umar Zai  November 13, 2023
Exploring the Landscape of Popular Cloud Computing Startup Companies Cloud computing has revolutionised the way businesses operate, providing scalable and…
WordPress Blog PlugIns
Umar Zai  
Exploring the best WordPress blog plugins for maximum impact In the dynamic world of blogging, the choice of the best…
AI Language Models
Umar Zai  
Exploring Progress and Obstacles: Delving into the Influence of AI Language Models on Society In the ever-evolving landscape of artificial…
Latest Tweet
No tweets found.